CSE NoticeBard presents the list of the Top 7 Java Cheatsheet Resources for Beginners. Check the most commonly used Java features and APIs below!
About Java Cheatsheet
A Java cheat sheet is a concise and quick reference guide that provides essential information, syntax, and examples related to Java programming. This is one of the necessary resources for programmers of all levels to efficiently refresh their knowledge of Java concepts without needing extensive documentation.
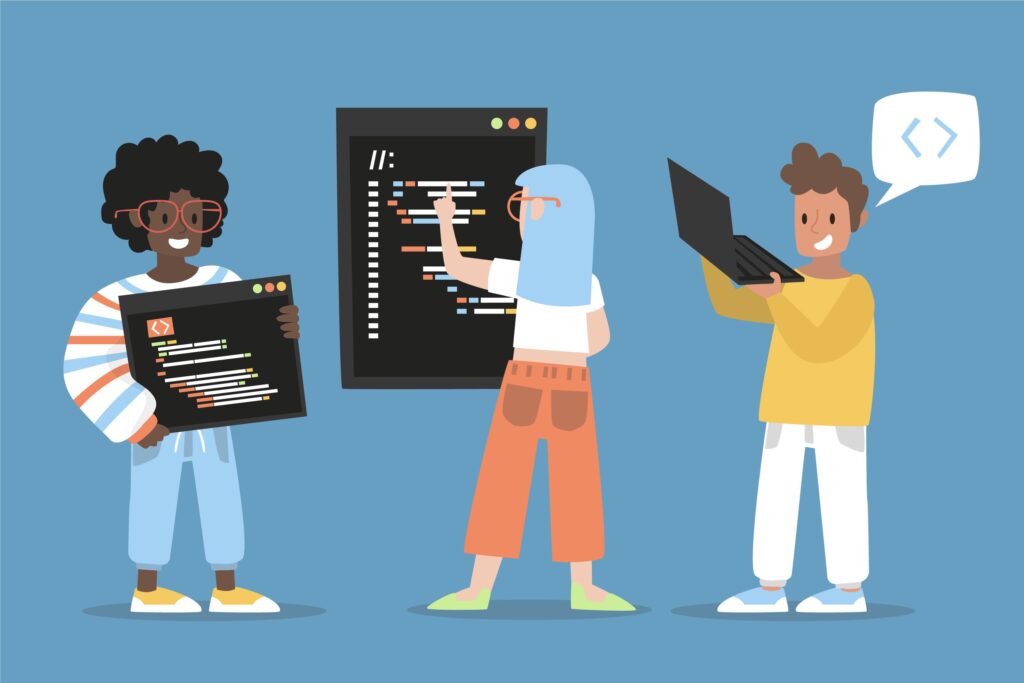
Topics Covered in Java Cheat Sheet
- Basic Syntax: Including how to declare variables, data types, operators, and comments.
- Control Structures: If statements, switch statements, loops (for, while, do-while), and conditional operators.
- Data Types and Variables: Covering primitive data types (int, double, char, boolean) and declaring variables.
- Arrays and Collections: How to declare and use arrays, ArrayLists, and other data structures.
- Functions (Methods): Syntax for declaring and calling methods, including parameters and return types.
- Object-Oriented Programming: Classes, objects, constructors, access modifiers, and inheritance.
- Exception Handling: Try-catch blocks, throwing and catching exceptions, and the Exception hierarchy.
- Input/Output: Reading from and writing to the console, files, and streams.
- Strings: String manipulation methods, concatenation, and formatting.
- Packages and Imports: Organizing code into packages and importing classes.
- Modifiers: Access modifiers (public, private, protected), static, final, and abstract.
- Keywords: A list of Java keywords and their meanings.
- Memory Management: Stack vs. heap memory, garbage collection, and memory leaks.
- Commonly Used Classes: Brief explanations of Scanner, Math, and String classes.
- Date and Time: Work with dates, times, and the DateTime API.
Java Basic Codes
// Basic Structure
public class MyClass {
public static void main(String[] args) {
// Your code here
}
}
// Variables and Data Types
int myInt = 10;
double myDouble = 3.14;
char myChar = 'A';
String myString = "Hello";
boolean myBool = true;
// Control Structures
if (condition) {
// Code to execute if condition is true
} else if (anotherCondition) {
// Code to execute if anotherCondition is true
} else {
// Code to execute if none of the conditions are true
}
for (int i = 0; i < 5; i++) {
// Code to repeat in each iteration
}
while (condition) {
// Code to execute while condition is true
}
// Arrays
int[] intArray = {1, 2, 3, 4, 5};
String[] stringArray = new String[3];
// Functions (Methods)
public returnType methodName(parameterType parameter) {
// Method body
return returnValue;
}
// Object-Oriented Programming
class MyClass {
private int myField; // Field or instance variable
public MyClass(int value) { // Constructor
myField = value;
}
public void myMethod() { // Method
// Code here
}
public int getMyField() { // Getter method
return myField;
}
}
// Inheritance
class ChildClass extends ParentClass {
// Additional fields and methods
}
// Exception Handling
try {
// Code that might throw an exception
} catch (ExceptionType e) {
// Code to handle the exception
} finally {
// Code that executes regardless of whether an exception occurred
}
// Input/Output
import java.util.Scanner;
Scanner scanner = new Scanner(System.in);
int userInput = scanner.nextInt();
System.out.println("User input: " + userInput);
// Libraries
import java.util.ArrayList;
import java.util.HashMap;
ArrayList list = new ArrayList<>();
HashMap map = new HashMap<>();
// Basic Input/Output
System.out.print("This is a message without a line break");
System.out.println("This is a message with a line break");
// Comments
// This is a single-line comment
/*
This is a
multi-line comment
*/
Java Language Basics Tutorials
Numerous tutorials are available in the public domain for novices to learn JAVA from scratch. However, if you are looking for authentic domains, check the links below directly from Java officials. Here we go,
- Creating Variables and Naming Them
- Creating Primitive Type Variables in Your Programs
- Creating Arrays in Your Programs
- Using the Var Type Identifier
- Using Operators in Your Programs
- Summary of Operators
- Expressions, Statements and Blocks
- Control Flow Statements
- Branching with Switch Statements
- Branching with Switch Expressions
Java Operators
Operator Type | Operators |
Arithmetic operators | +,-,/,*,% |
Relational operators | <, >, <=, >=,==, != |
Logical operators | && , || |
Assignment operator | =, +=, −=, ×=, ÷=, %=, &=, ^=, |=, <<=, >>=, >>>= |
Increment and Decrement operator | ++ , – – |
Special operators | . (dot operator to access methods of class) |
Bitwise operators | ^, &, | |
Conditional operators | ?: |
Java Keywords
Keyword | Function |
abstract | The abstract keyword may modify a class or a method |
boolean | The abstract a keyword may modify a class or a method |
break | The break keyword is used to prematurely exit a for, while, or do a loop or to mark the end of a case block in a switch statement. |
byte | The byte is a primitive type. This keyword is used to declare a variable that can hold 8-bit data values. |
case | The case is used to label each branch in a switch statement. |
catch | The boolean keyword is used to declare a variable as a boolean type. It can hold True and false values only. |
char | The continue the keyword is used to skip to the next iteration of a for, while, or do loop. |
class | The class keyword is used to declare a new Java class, which is a collection of related variables and/or methods. |
continue | The default the keyword is used to label the default branch in a switch statement. |
default | The catch the keyword is used to define exception-handling blocks in try−catch or try−catch−finally statements. |
do | The do keyword specifies a loop whose condition is checked at the end of each iteration |
double | The double keyword is used to declare a variable that can hold 64-bit floating-point number. double is a Java primitive type. |
else | The else keyword is always used in association with the if keyword in an if−else statement. The else clause is optional and is executed if the if condition is false |
extends | The extends keyword is used in a class or interface declaration to indicate that the class or interface being declared is a subclass of the class or interface whose name follows the extends keyword. |
false | The false keyword represents one of the two legal values for a boolean variable. |
final | The final keyword may be applied to a class, indicating the class may not be extended (subclassed). The final keyword may be applied to a method, indicating the method may not be overridden in any subclass. |
finally | The finally keyword is used to define a block that is always executed in a try−catch−finally statement. A ‘finally’ block typically contains cleanup code that recovers from the partial execution of a try block. |
float | The float keyword is used to declare a variable that can hold a 32-bit floating-point number; float is a Java primitive type. |
for | The for keyword specifies a loop whose condition is checked before each iteration. |
if | The if keyword indicates the conditional execution of a block. The condition must evaluate to a boolean value. It executes the if block if the condition is true. |
List of Top 7 Java Cheatsheets Resources for Beginners
Java Programming Cheatsheet by Princeton University
Java Cheat Sheet by IterviewBit
Java Cheatsheet by GeeksforGeeks
Java Cheat Sheet by CodeWithHarry
Java Cheat Sheet by GitHub
Java Cheat Sheet by Programming with Mosh
Java Cheat Sheet by Hackr io
For more updates on Data Science, AWS, Python and Java Cheatsheet Resources, stay connected with the cse.noticebard.com!
The post has been updated on November 21, 2024!